Getting Started
Access powerful onchain data curated by the Zapper Protocol with a GraphQL API.
Portfolio Data
A set of portfolio queries to fetch Tokens, NFTs, App Balances, Portfolio Totals, and Claimables.
Token Prices & Charts
A price for every token that has an onchain market, including historical data.
Human-Readable Transactions
Build rich transaction views with simple and human-friendly descriptions.
Onchain Search
Comprehensive search results across multiple chains and entity types with a single query.
NFTs
Rich NFT metadata with media, traits, holders, valuations and more.
Account Identity
Surface identity primitives such as avatars, ENS, Basenames, Farcaster, and Lens.
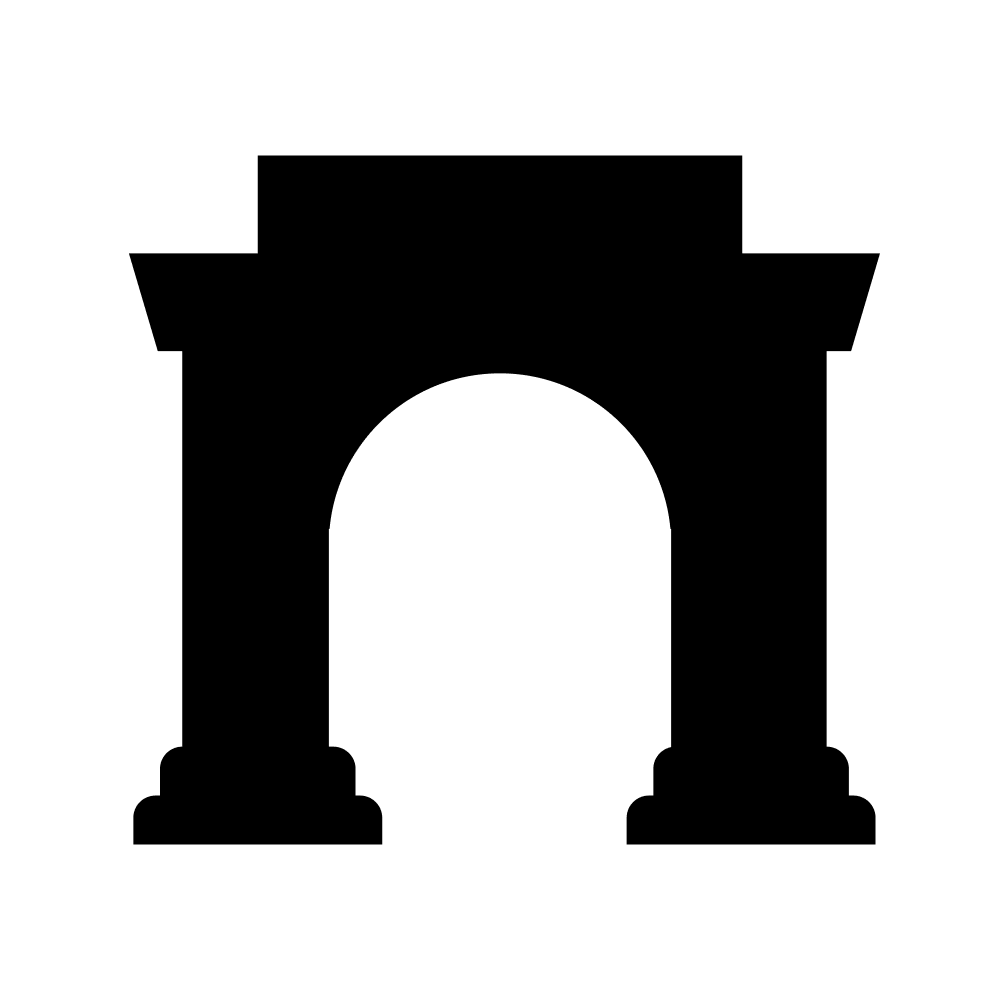
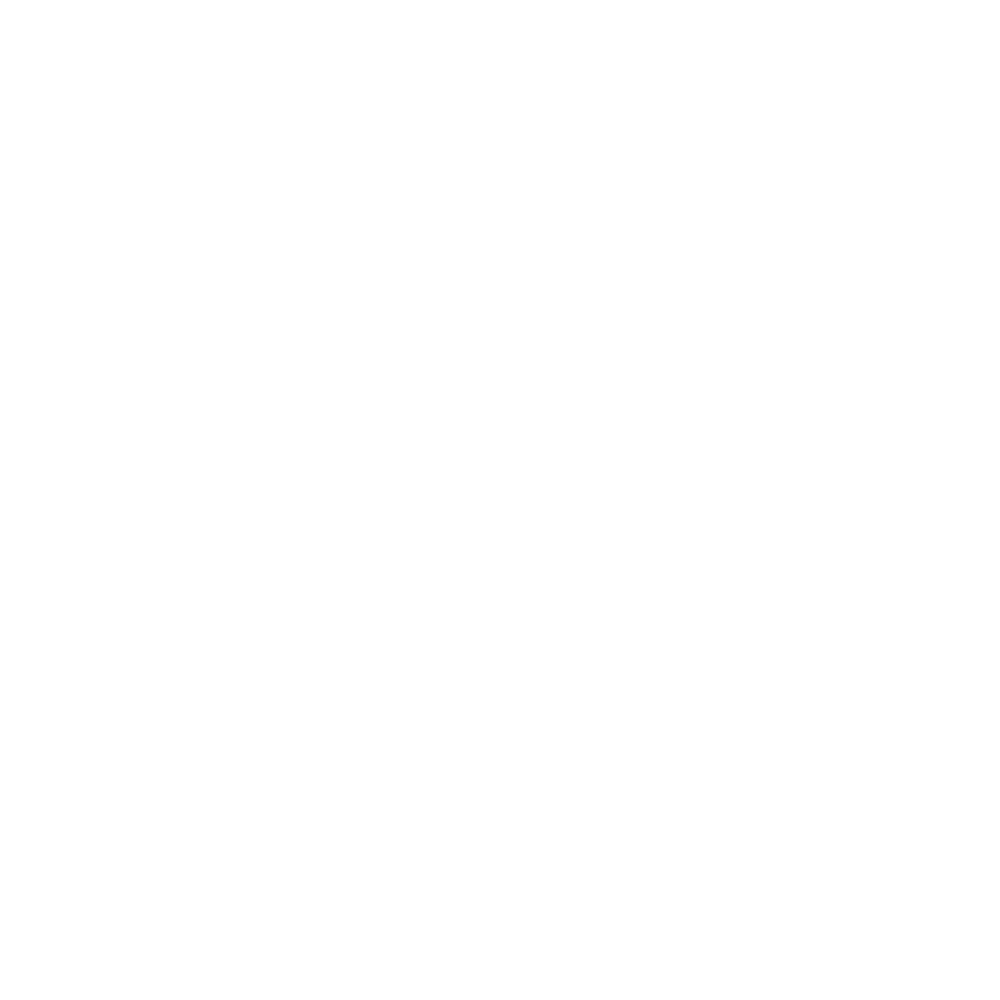
Farcaster
Complete onchain portfolio & transaction history for any Farcaster user
Quickstart
1) Get an API key
2) Start building
Below are examples for working with the Zapper API across different languages and frameworks. Each example shows how to fetch portfolio data for provided addresses, across the chosen chains. Additionally, our API Sandbox lets you try our endpoints in one click.
If you are working with an AI agent, we also recommend providing our customized prompt to start building efficiently.
- React
- Node.js
- cURL
- Python
- Ruby
Setup
- Create new React project:
npm create vite@latest my-app -- --template react-ts
- Navigate to your new directory :
cd my-app
- Install dependencies:
npm install @apollo/client graphql
- Replace
src/App.tsx
with code below - Replace YOUR_API_KEY with your actual key
- Run:
npm run dev
New to React? Get started with Create React App or Next.js.
import { ApolloClient, InMemoryCache, createHttpLink, gql, useQuery, ApolloProvider } from '@apollo/client';
import { setContext } from '@apollo/client/link/context';
interface TokenNode {
balance: number;
balanceRaw: string;
balanceUSD: number;
symbol: string;
name: string;
}
interface TokenEdge {
node: TokenNode;
}
interface PortfolioV2Data {
portfolioV2: {
tokenBalances: {
byToken: {
edges: TokenEdge[];
}
}
};
}
// Set up Apollo Client
const httpLink = createHttpLink({
uri: 'https://public.zapper.xyz/graphql',
});
const API_KEY = 'YOUR_API_KEY';
const authLink = setContext((_, { headers }) => {
return {
headers: {
...headers,
'x-zapper-api-key': API_KEY,
},
};
});
const client = new ApolloClient({
link: authLink.concat(httpLink),
cache: new InMemoryCache(),
});
const PortfolioV2Query = gql`
query PortfolioV2($addresses: [Address!]!, $networks: [Network!]) {
portfolioV2(addresses: $addresses, networks: $networks) {
tokenBalances {
byToken {
edges {
node {
balance
balanceRaw
balanceUSD
symbol
name
}
}
}
}
}
}
`;
function Portfolio() {
const { loading, error, data } = useQuery<PortfolioV2Data>(PortfolioV2Query, {
variables: {
addresses: ['0x3d280fde2ddb59323c891cf30995e1862510342f'],
networks: ['ETHEREUM_MAINNET'],
},
});
if (loading) return <div>Loading...</div>;
if (error) return <div>Error: {error.message}</div>;
if (!data) return <div>No data found</div>;
return (
<div className="p-4">
{data.portfolioV2.tokenBalances.byToken.edges.map((edge, index) => (
<div key={`${edge.node.symbol}-${index}`} className="mb-4 p-4 border rounded">
<p>Token: {edge.node.name}</p>
<p>Symbol: {edge.node.symbol}</p>
<p>Balance: {edge.node.balance}</p>
<p>Raw Balance: {edge.node.balanceRaw}</p>
<p>Value (USD): ${edge.node.balanceUSD.toFixed(2)}</p>
</div>
))}
</div>
);
}
function App() {
return (
<ApolloProvider client={client}>
<Portfolio />
</ApolloProvider>
);
}
export default App;
Setup
- Create new directory and enter it
- Run
npm init -y
- Install axios:
npm install axios
- Create
index.js
with code below - Replace YOUR_API_KEY
- Run:
node index.js
New to Node.js? Get started with the official guide.
const axios = require('axios');
const API_KEY = 'YOUR_API_KEY';
const query = `
query PortfolioV2($addresses: [Address!]!, $networks: [Network!]) {
portfolioV2(addresses: $addresses, networks: $networks) {
tokenBalances {
byToken {
edges {
node {
balance
balanceRaw
balanceUSD
symbol
name
}
}
}
}
}
}
`;
async function fetchPortfolio() {
try {
const response = await axios({
url: 'https://public.zapper.xyz/graphql',
method: 'post',
headers: {
'Content-Type': 'application/json',
'x-zapper-api-key': API_KEY,
},
data: {
query,
variables: {
addresses: ['0x3d280fde2ddb59323c891cf30995e1862510342f'],
networks: ['ETHEREUM_MAINNET'],
},
},
});
if (response.data.errors) {
throw new Error(`GraphQL Errors: ${JSON.stringify(response.data.errors)}`);
}
return response.data.data;
} catch (error) {
console.error('Error fetching portfolio:', error.message);
if (error.response) {
console.error('Response data:', error.response.data);
}
throw error;
}
}
// Example usage
(async () => {
try {
const portfolioData = await fetchPortfolio();
console.log("Portfolio data:");
const tokens = portfolioData.portfolioV2.tokenBalances.byToken.edges;
tokens.forEach((edge, index) => {
const token = edge.node;
console.log(`\n--- Token ${index + 1} ---`);
console.log(`Name: ${token.name}`);
console.log(`Symbol: ${token.symbol}`);
console.log(`Balance: ${token.balance}`);
console.log(`Raw Balance: ${token.balanceRaw}`);
console.log(`Value (USD): $${token.balanceUSD.toFixed(2)}`);
});
} catch (error) {
console.error('Failed to fetch portfolio:', error.message);
process.exit(1);
}
})();
Setup
cURL is usually pre-installed on Unix-based systems. For Windows, download it here.
API_KEY="YOUR_API_KEY"
Run the cURL command using your API key:
curl --location 'https://public.zapper.xyz/graphql' \
--header 'Content-Type: application/json' \
--header "x-zapper-api-key: $API_KEY" \
--data '{"query":"query PortfolioV2($addresses: [Address!]!, $networks: [Network!]) { portfolioV2(addresses: $addresses, networks: $networks) { tokenBalances { byToken { edges { node { balance balanceRaw balanceUSD symbol name } } } } } }","variables":{"addresses":["0x3d280fde2ddb59323c891cf30995e1862510342f"],"networks":["ETHEREUM_MAINNET"]}}'
Setup
- Create new directory:
mkdir python-portfolio && cd python-portfolio
- Create virtual environment:
python3 -m venv venv
- Activate virtual environment:
source venv/bin/activate
- Install requests:
pip install requests
- Create
portfolio.py
with code below - Replace YOUR_API_KEY
- Run:
python portfolio.py
New to Python? Get started with the official tutorial.
import requests
from typing import Dict, Any
API_KEY = 'YOUR_API_KEY'
query = """
query PortfolioV2($addresses: [Address!]!, $networks: [Network!]) {
portfolioV2(addresses: $addresses, networks: $networks) {
tokenBalances {
byToken {
edges {
node {
balance
balanceRaw
balanceUSD
symbol
name
}
}
}
}
}
}
"""
def fetch_portfolio() -> Dict[str, Any]:
try:
response = requests.post(
'https://public.zapper.xyz/graphql',
headers={
'Content-Type': 'application/json',
'x-zapper-api-key': API_KEY
},
json={
'query': query,
'variables': {
'addresses': ['0x3d280fde2ddb59323c891cf30995e1862510342f'],
'networks': ['ETHEREUM_MAINNET']
}
},
timeout=30
)
response.raise_for_status()
data = response.json()
if 'errors' in data:
raise ValueError(f"GraphQL Errors: {data['errors']}")
return data['data']
except requests.RequestException as e:
print(f"Request failed: {e}")
raise
except ValueError as e:
print(f"Data validation failed: {e}")
raise
except Exception as e:
print(f"Unexpected error: {e}")
raise
if __name__ == "__main__":
try:
portfolio_data = fetch_portfolio()
print("Portfolio data:")
tokens = portfolio_data['portfolioV2']['tokenBalances']['byToken']['edges']
for i, edge in enumerate(tokens):
token = edge['node']
print(f"\n--- Token {i + 1} ---")
print(f"Name: {token['name']}")
print(f"Symbol: {token['symbol']}")
print(f"Balance: {token['balance']}")
print(f"Raw Balance: {token['balanceRaw']}")
print(f"Value (USD): ${token['balanceUSD']:.2f}")
except Exception as e:
print(f"Failed to fetch portfolio: {e}")
Setup
- Install Ruby if not installed:
brew install ruby
(macOS) or follow Ruby installation guide - Create new directory:
mkdir ruby-portfolio && cd ruby-portfolio
- Create
portfolio.rb
with code below - Replace YOUR_API_KEY
- Run:
ruby portfolio.rb
New to Ruby? Get started with Ruby in 20 minutes.
require 'net/http'
require 'uri'
require 'json'
API_KEY = 'YOUR_API_KEY'
query = <<-GRAPHQL
query PortfolioV2($addresses: [Address!]!, $networks: [Network!]) {
portfolioV2(addresses: $addresses, networks: $networks) {
tokenBalances {
byToken {
edges {
node {
balance
balanceRaw
balanceUSD
symbol
name
}
}
}
}
}
}
GRAPHQL
def fetch_portfolio
uri = URI('https://public.zapper.xyz/graphql')
http = Net::HTTP.new(uri.host, uri.port)
http.use_ssl = true
http.read_timeout = 30
http.open_timeout = 30
request = Net::HTTP::Post.new(uri)
request['Content-Type'] = 'application/json'
request['x-zapper-api-key'] = API_KEY
request.body = {
query: query,
variables: {
addresses: ['0x3d280fde2ddb59323c891cf30995e1862510342f'],
networks: ['ETHEREUM_MAINNET']
}
}.to_json
response = http.request(request)
unless response.is_a?(Net::HTTPSuccess)
raise "HTTP Request failed: #{response.code} - #{response.message}"
end
data = JSON.parse(response.body)
if data['errors']
raise "GraphQL Errors: #{data['errors']}"
end
data['data']
rescue JSON::ParserError => e
raise "Failed to parse JSON response: #{e.message}"
rescue StandardError => e
raise "Error fetching portfolio: #{e.message}"
end
begin
portfolio = fetch_portfolio
puts "Portfolio data:"
tokens = portfolio['portfolioV2']['tokenBalances']['byToken']['edges']
tokens.each_with_index do |edge, index|
token = edge['node']
puts "\n--- Token #{index + 1} ---"
puts "Name: #{token['name']}"
puts "Symbol: #{token['symbol']}"
puts "Balance: #{token['balance']}"
puts "Raw Balance: #{token['balanceRaw']}"
puts "Value (USD): $#{format('%.2f', token['balanceUSD'])}"
end
rescue StandardError => e
puts "Failed to fetch portfolio: #{e.message}"
exit 1
end
3) Buy Credits
Zapper API uses a credit system to manage how many queries an API key can perform. Each query made costs a certain amount of credits which can be found by visiting Pricing.
Track API usage and purchase additional credits via the API Dashboard.
If you are a client of the legacy REST API, you have access to the new GraphQL endpoints with your existing API key, after signing in to the new Dashboard with the email associated with your existing account. Please contact us at [email protected] to get your purchased points migrated to the new GraphQL API.